Java Barcode Generator for Codabar
Java Codabar Generation package to generate Codabar linear barcodes in Java applications.
- Easily create high-quality linear Codabar barcodes images of AWT, JPEG, and GIF formats in Java, Java Class, J2EE applications.
- JSP, Java Servlet and J2EE web projects are available for Codabar barcode generation.
- Compatible with the latest ISO barcode Standard.
- Servlets, Applets, JavaBean and class library are provided in each Java Codabar generation package.
- Royalty free with a permanent license by Avapose.
- Single jar program packages are developed for each Codabar barcode symbology.
- Compatible with JDK 4.0 and above
Codabar Symbology Overview
Codabar information:
Codabar: is also known as Ames Code, NW-7, USD-4, Code 2 of 7, is a numeric-only linear barcode type without a check digit and now is widely used in U.S. blood banks, photo labs, and FedEx.
Available encoding digits:
Numeric digits 0 to 9,
Special digits: - $ : / +
Variable data length
The structure of a Codabar:
1. One of four possible start characters (A, B, C, or D).
2. A narrow, inter-character space.
3. The data of the message with a narrow inter-character space between each character.
4. One of four possible stop characters (A, B, C, or D).
|
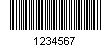 |
Java Codabar Generator Implementation to Generate Codabar
1.Generate barcodes in Java class by changing of barcode properties.
// Create Java barcode object
Codbar barcode = new Codabar();
// Set barcode data text to encode
barcode.setData("1234567");
// Generate barcode & print into Graphics2D object
barcode.drawBarcode("Java Graphics2D object");
// Generate barcode & encode into GIF format
barcode.drawBarcode("C://barcode- codabar.gif");
// Generate barcode & encode into JPEG format
barcode.drawBarcode("C://barcode- codabar.jpg");
2. Generate & encode barcodes to EPS by changing the values concerned.
// Create Java Codabar object
Codabar barcode = new Codabar();
// Set Codabar data text to encode
barcode.setData("1234567");
// Generate Codabar barcode & encode into EPS
barcode.drawBarcode2EPS("C://barcode-codabar.eps");
3. Generate & encode barcodes in html or jsp pages.
1. Copy barcode folder and its contents from demo package to Apache Tomcat.
2. Start Apache Tomcat, go to http://YourDomain:Port/barcode/barcode?DATA=1234567&TYPE=CODABAR
3. Insert an image tag (img) into your page. For example,
<imgsrc="http://YourDomain:Port/barcode/barcode?DATA=1234567&TYPE=CODABAR" />
4. Two ways to set the width and height of generated barcode Codabar image:
1. Set the values of the barcodeWidth and barcodeHeight properties.
or
2. Set X (bar module width) and Y (bar module height) values
Codabar Property Settings in Java Barcode Generator
Codabar Basic Settings
Properties |
Value |
Comments
|
Property |
URL |
Options |
Default |
data |
DATA |
(Data to encode in the Codabar) |
"" |
Codabar value to encode
Codabar Character Set:
- Digits:0, 1, 2, 3, 4, 5, 6, 7, 8, 9;
- - (Dash), $ (Dollar), : (Colon), / (Slash), . (Point), + (Plus)
|
addCheckSum |
ADD-CHECK-SUM |
(Data to encode in the Codabar) |
false |
addCheckSum property is not applied here. Codabar does not require any checksum.
|
Codabar Special Settings
Properties |
Value |
Comments |
Property |
URL |
Options |
Default |
startChar
|
START
-CHAR |
A, B, C, or D
|
A |
Codabar start char, valid values: CodabarStartStopChar.A, B, C, D
|
stopChar
|
STOP-CHAR |
A, B, C, or D
|
A |
Codabar stop char, valid values: CodabarStartStopChar.A, B, C, D
|
N
|
N |
float
|
2.of |
Wide/narrow ratio, 2.0 - 3.0 inclusive, default is 2.
|
Codabar Text Settings
Properties |
Value |
Comments |
Property |
URL |
Options |
Default |
ShowText
|
SHOW-TEXT |
true or false |
true |
Show text underneath the barcode
|
TextColor
|
TEXT-COLOR |
Color
|
black |
Color of the shown text
|
TextFont
|
|
Font
|
new Font("Arial",Font.PLAIN,11) |
The font, font style and font size of the text
|
TextMargin
|
|
float
|
6 |
The space between the text and the barcode symbol
|
Codabar Size Settings
Properties |
Value |
Comments |
Property |
URL |
Options |
Default |
rotate
|
ROTATE |
Degree0, 90, 180, 270
|
0 (Barcode.ROTATE) |
Rotate the Angle of the Codabar images
|
autoResize
|
AUTO-RESIZE |
true or false
|
false |
Auto resize the generated barcode image
|
barcodeHeight
|
BARCODE-HEIGHT |
float
|
0 |
Barcode image Height
|
barcodeWidth
|
BARCODE-WIDTH |
float
|
0 |
Barcode image Width
|
bottomMargin
|
BOTTOM-MARGIN |
float |
0 |
Barcode image bottom margin size
|
leftMargin |
LEFT-MARGINE |
float |
0 |
Barcode image left margin size
|
rightMargin |
RIGHT-MARGIN |
float |
0 |
Barcode image right margin size.
|
topMargin |
TOP-MARGIN |
float |
0 |
Barcode image Top margin size
|
barAlignment |
BARALIGMENT |
int |
1(center) |
Barcode horizontal alignment inside the image. 0: left, 1: center, 2: right.
|
uom
|
UOM |
Pixel, Cm, or Inch |
Pixel |
Unit of Measure for all numeric properties
|
X |
X |
float |
2 |
Width of barcode bar module (narrow bar), default is 2 pixel
|
Y |
Y |
float |
75 |
Height of barcode bar module, default is 75 pixel
|
Codabar Color Settings
Properties |
Value |
Comments |
Property |
URL |
Options |
Default |
backColor
|
BACK
-COLOR |
Color |
white |
Barcode image background color
|
foreColor
|
FORE
-COLOR |
Color
|
black |
Barcode image foreground color
|
Codabar Image Settings
Properties |
Value |
Comments |
Property |
URL |
Options |
Default |
resolution
|
RESOLUTION |
int |
72 |
Resolution of Codabar Image, Dots Per Inch
|
ImageFormat
|
IMAGE-FORMAT |
Memory Bmp, Bmp, Emf, Wmf, Gif, Jpeg, Png, Tiff, Exif, or Icon
|
Gif |
Barcode image format
|
Method
// generate barcode and output to OutputStream object
public boolean drawBarcode(OutputStream outputStream) throws Exception
// generate barcode into a new BufferedImage object
public BufferedImage drawBarcode() throws Exception
/*
Use this method to generate barcode, and save into gif or jpeg files
1. to save into gif file, filename ends with ".gif", like "c:\\barcode.gif"
2. to save into jpeg file, filename ends with ".jpg", like "c:\\barcode.jpg"
*/
public byte[] drawBarcodeToBytes() throws Exception
public boolean drawBarcode(String imageFile) throws Exception
// Generate barcode and save into EPS file, the filename must ends with ".eps"
public void drawBarcode2EPS(String filename) throws Exception
// Generate barcode on Graphics2D object within certain area
public void drawBarcode(Graphics2D g, Rectangle2D rectangle) throws Exception
|
|